4. Drawing graphics
To create graphics for our games we will use Raylib along with my RLZero library. You will find the documentation on the websites useful!
4.1. Installing the graphics library
Installing a Python package varies depending on what Python editor or IDE you are using. Here is how you do it if you are using the Mu editor.
Run Mu. Click Mode and select Python3.
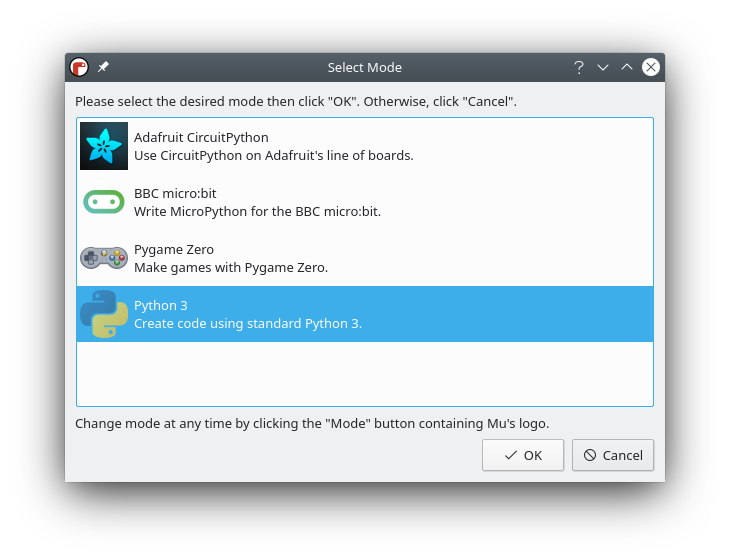
Then click the small gadget icon in the bottom right hand corner of the window. Click third party packages. Type
rlzero>=0.3<0.4
into the box. Click OK. The library will download.
If you are not using Mu you can install from the command line like this:
python3 -m pip install 'rlzero>=0.3<0.4'
4.2. RLZero and Raylib
Raylib is a graphics library. RLZero adds some extra functions to Raylib to make it easier to use. Once you have installed RLZero you are free to use all the functions of Raylib- you don’t have to stick to the RLZero functions.
4.3. Pixels
The smallest square that can be displayed on a monitor is called a pixel. This diagram shows a close-up view of a window that is 40 pixels wide and 40 pixels high. At normal size you will not see the grid lines.
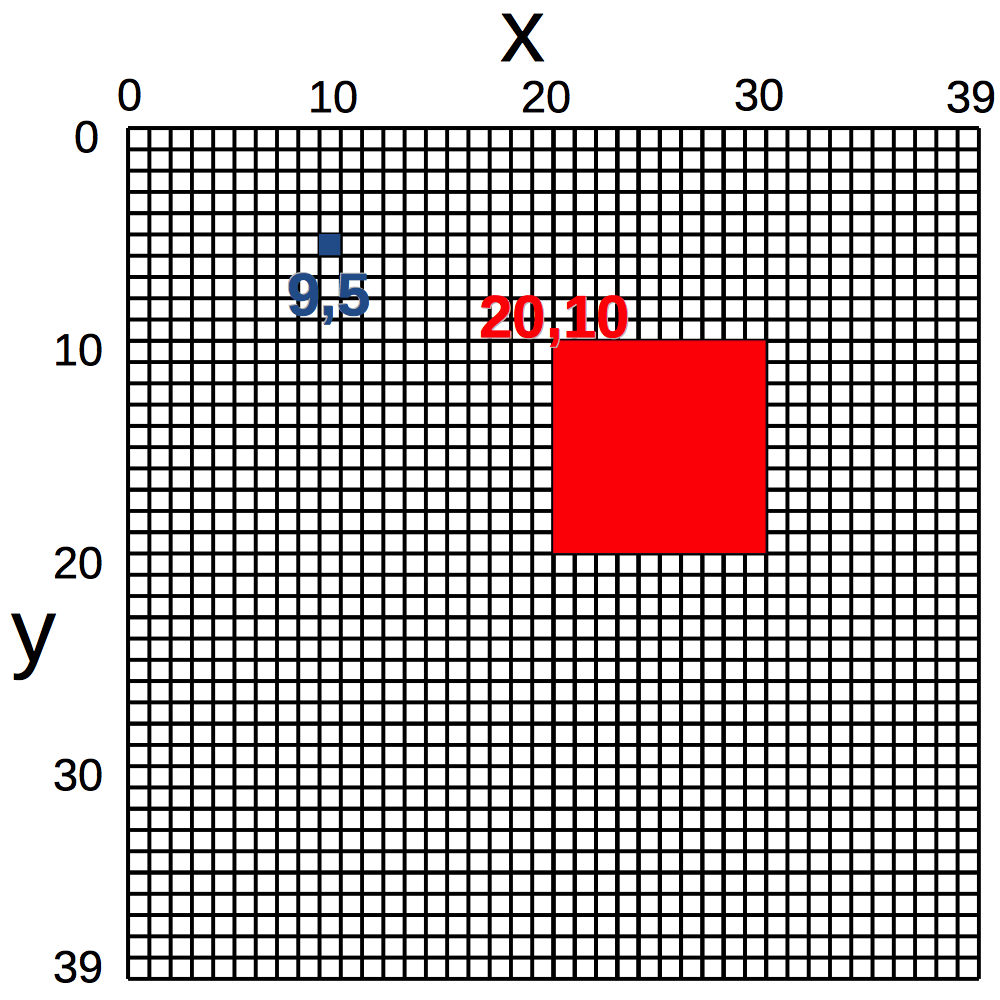
We can refer to any pixel by giving two co-ordinates, (x,y) . Make sure you understand co-ordinates before moving on because everything we do will use them. (In maths this called a ‘Cartesian coordinate system’.)
Now type in Program 4.1 and run it, to see a pixel. It’s very small, so look carefully!
1from rlzero import *
2
3WIDTH = 500 # What are these units? What if we change them?
4HEIGHT = 500 # What if we delete this line?
5
6def draw():
7 clear()
8 draw_pixel(250, 250, RED)
9
10run()
Exercise
Make this pixel blue.
4.4. Lines and circles
1from rlzero import *
2
3WIDTH = 500
4HEIGHT = 500
5
6def draw():
7 draw_circle_lines(250, 250, 50, WHITE)
8 draw_circle(250, 100, 50, RED)
9 draw_line(150, 20, 150, 450, PURPLE)
10 draw_line(150, 20, 350, 20, PURPLE)
11
12run()
Exercise
Finish drawing this picture
Exercise
Draw your own picture.
4.5. Moving rectangles
To make things move we need to add the special update()
function. We
don’t need to write our own loop because RLZero calls this
function for us in its own loop, over and over, many times per second.
1from rlzero import *
2
3WIDTH = 500
4HEIGHT = 500
5
6box = Rectangle(20, 20, 50, 50)
7
8
9def draw():
10 draw_rectangle_rec(box, RED)
11
12def update():
13 box.x = box.x + 2
14 if box.x > WIDTH:
15 box.x = 0
16
17run()
Exercise
Make the box move faster.
Exercise
Make the box move in different directions.
Exercise
Make two boxes with different colours.
4.6. Sprites
Sprites are very similar to boxes, but are loaded from a png or jpg image file.
RLZero has one such image built-in,
alien.png
. If you want to use other images you must create them
and place the files in the same directory as your program.
We are going to store the sprite in a variable so we can refer to it again when we want to draw it. We have called this variable alan. We could create more sprites using the same image, but we would have to use different variable names.
You could use Microsoft Paint which comes with Windows but I recommend you download and install Krita.
1from rlzero import *
2
3WIDTH = 500
4HEIGHT = 500
5
6alan = Sprite('alien.png')
7alan.x = 50
8alan.y = 100
9
10
11def draw():
12 alan.draw()
13
14
15def update():
16 alan.x += 1
17 if alan.x > WIDTH:
18 alan.x = 0
19
20run()
21
Exercise
Draw or download your own image to use instead of alien.
4.7. Background image
We are going to add a background image to Program 4.4
You must create or download a picture to use a background. Save it as
background.png
in the folder with your program. It should be the
same size as the window, 500×500 pixels and it must be in .png
format.
1from rlzero import *
2
3WIDTH = 500
4HEIGHT = 500
5
6alan = Sprite('alien.png')
7alan.x = 0
8alan.y = 50
9
10background = Sprite('background.png')
11
12def draw():
13 background.draw()
14 alan.draw()
15
16
17def update():
18 alan.x += 2
19 if alan.x > WIDTH:
20 alan.x = 0
21
22run()
23
Exercise
Create a picture to use a background. Save it as background.png. Run the program.
4.8. Keyboard input
Alan moves when you press the cursor keys.
1from rlzero import *
2
3alan = Sprite('alien.png')
4alan.pos = (0, 50)
5
6def draw():
7 alan.draw()
8
9def update():
10 if keyboard.right:
11 alan.x = alan.x + 2
12 elif keyboard.left:
13 alan.x = alan.x - 2
14
15run()
Exercise
Make Alan move up and down as well as left and right.
Exercise
Use the more concise += operator to change the alien.x value (see Section 2.11).
Exercise
Use the or operator to allow WASD keys to move the alien in addition to the cursor keys (see Program 2.8).